✅P190_商城业务-检索服务-面包屑导航
大约 2 分钟
一、面包屑导航
页面展示
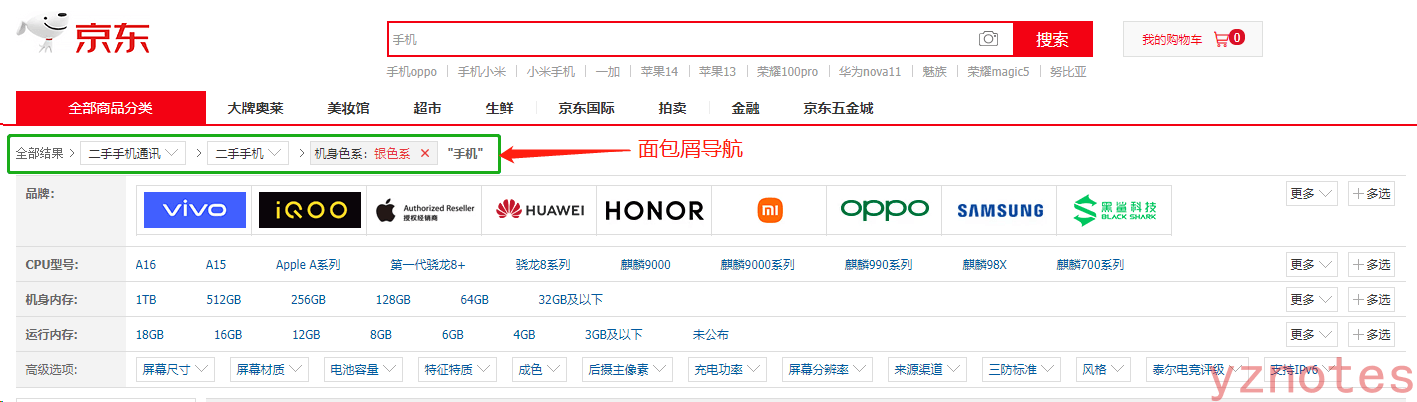
完成”商城业务-检索服务-条件筛选联动“后,再看效果!
二、面包屑导航Vo
封装导航栏VO
cfmall-search/src/main/java/com/gyz/cfmall/search/vo/SearchResult.java
//省略其它代码...
private List<NavVo> navs;
@Data
public static class NavVo {
private String navName;
private String navValue;
private String link;
}
三、封装面包屑导航数据
com.gyz.cfmall.search.service.impl.MallSearchServiceImpl#buildSearchResult
//6、构建面包屑导航
if (param.getAttrs() != null && param.getAttrs().size() > 0) {
List<SearchResult.NavVo> collect = param.getAttrs().stream().map(attr -> {
//1、分析每一个attrs传过来的参数值
SearchResult.NavVo navVo = new SearchResult.NavVo();
String[] s = attr.split("_");
//封装属性值
navVo.setNavValue(s[1]);
//封装属性名
R r = productFeignService.attrInfo(Long.parseLong(s[0]));
if (r.getCode() == 0) {
AttrResponseVo data = r.getData("attr", new TypeReference<AttrResponseVo>() {
});
navVo.setNavName(data.getAttrName());
} else {
navVo.setNavName(s[0]);
}
return navVo;
}).collect(Collectors.toList());
result.setNavs(collect);
}
四、获取属性名
属性名的获取要通过远程服务调用product服务进行查询
4.1 依赖管理
4.1.1 导入cloud的版本
cfmall-search/pom.xml
<spring-cloud.version>Greenwich.SR3</spring-cloud.version>
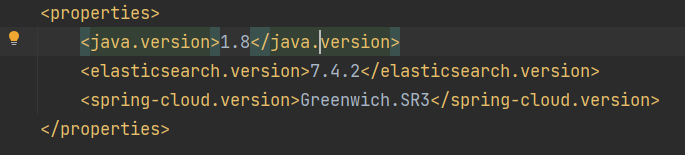
4.1.2 导入cloud依赖管理
cfmall-search/pom.xml
<dependencyManagement>
<dependencies>
<dependency>
<groupId>org.springframework.cloud</groupId>
<artifactId>spring-cloud-dependencies</artifactId>
<version>${spring-cloud.version}</version>
<type>pom</type>
<scope>import</scope>
</dependency>
</dependencies>
</dependencyManagement>
4.1.3 导入openfeign的依赖
<!--cfmall-search/pom.xml-->
<dependency>
<groupId>org.springframework.cloud</groupId>
<artifactId>spring-cloud-starter-openfeign</artifactId>
</dependency>
4.2 开启远程服务调用功能
添加@EnableFeignClients
注解,
com.gyz.cfmall.search.CfmallSearchApplication
@SpringBootApplication(exclude = DataSourceAutoConfiguration.class)
@EnableDiscoveryClient
@EnableFeignClients
public class CfmallSearchApplication {
public static void main(String[] args) {
SpringApplication.run(CfmallSearchApplication.class, args);
}
}
4.3 编写feign接口
com.gyz.cfmall.search.feign.ProductFeignService
@FeignClient("cfmall-product")
public interface ProductFeignService {
@GetMapping("/product/attr/info/{attrId}")
R attrInfo(@PathVariable("attrId") Long attrId);
}
4.4 返回数据格式处理
/**
* 利用fastjson进行反序列化
* com.gyz.common.utils.R#getData(java.lang.String, com.alibaba.fastjson.TypeReference<T>)
*/
public <T> T getData(String key, TypeReference<T> typeReference) {
//默认是map
Object data = get(key);
String jsonString = JSON.toJSONString(data);
T t = JSON.parseObject(jsonString, typeReference);
return t;
}
4.5 逻辑代码
cfmall-product/src/main/java/com/gyz/cfmall/product/controller/AttrController.java
/**
* 信息
*/
@RestController
@RequestMapping("product/attr")
public class AttrController {
@Autowired
private AttrService attrService;
@RequestMapping("/info/{attrId}")
public R info(@PathVariable("attrId") Long attrId) {
AttrRespVo respVo = attrService.getAttrInfo(attrId);
return R.ok().put("attr", respVo);
}
}
cfmall-product/src/main/java/com/gyz/cfmall/product/service/impl/AttrServiceImpl.java
@Override
public AttrRespVo getAttrInfo(Long attrId) {
//查询详细信息
AttrEntity attrEntity = this.getById(attrId);
//查询分组信息
AttrRespVo respVo = new AttrRespVo();
BeanUtils.copyProperties(attrEntity, respVo);
//判断是否是基本类型
if (attrEntity.getAttrType() == ProductConstant.AttrEnum.ATTR_TYPE_BASE.getCode()) {
//1、设置分组信息
AttrAttrgroupRelationEntity relationEntity = relationDao.selectOne
(new QueryWrapper<AttrAttrgroupRelationEntity>().eq("attr_id", attrId));
if (relationEntity != null) {
respVo.setAttrGroupId(relationEntity.getAttrGroupId());
//获取分组名称
AttrGroupEntity attrGroupEntity = attrGroupDao.selectById(relationEntity.getAttrGroupId());
if (attrGroupEntity != null) {
respVo.setGroupName(attrGroupEntity.getAttrGroupName());
}
}
}
//2、设置分类信息
Long catelogId = attrEntity.getCatelogId();
Long[] catelogPath = categoryService.findCatelogPath(catelogId);
respVo.setCatelogPath(catelogPath);
CategoryEntity categoryEntity = categoryDao.selectById(catelogId);
if (categoryEntity != null) {
respVo.setCatelogName(categoryEntity.getName());
}
return respVo;
}
cfmall-product/src/main/java/com/gyz/cfmall/product/vo/AttrRespVo.java
@Data
public class AttrRespVo extends AttrVo {
private String catelogName;
private String groupName;
private Long[] catelogPath;
}